Understanding JavaScript Classes for Creative Coding
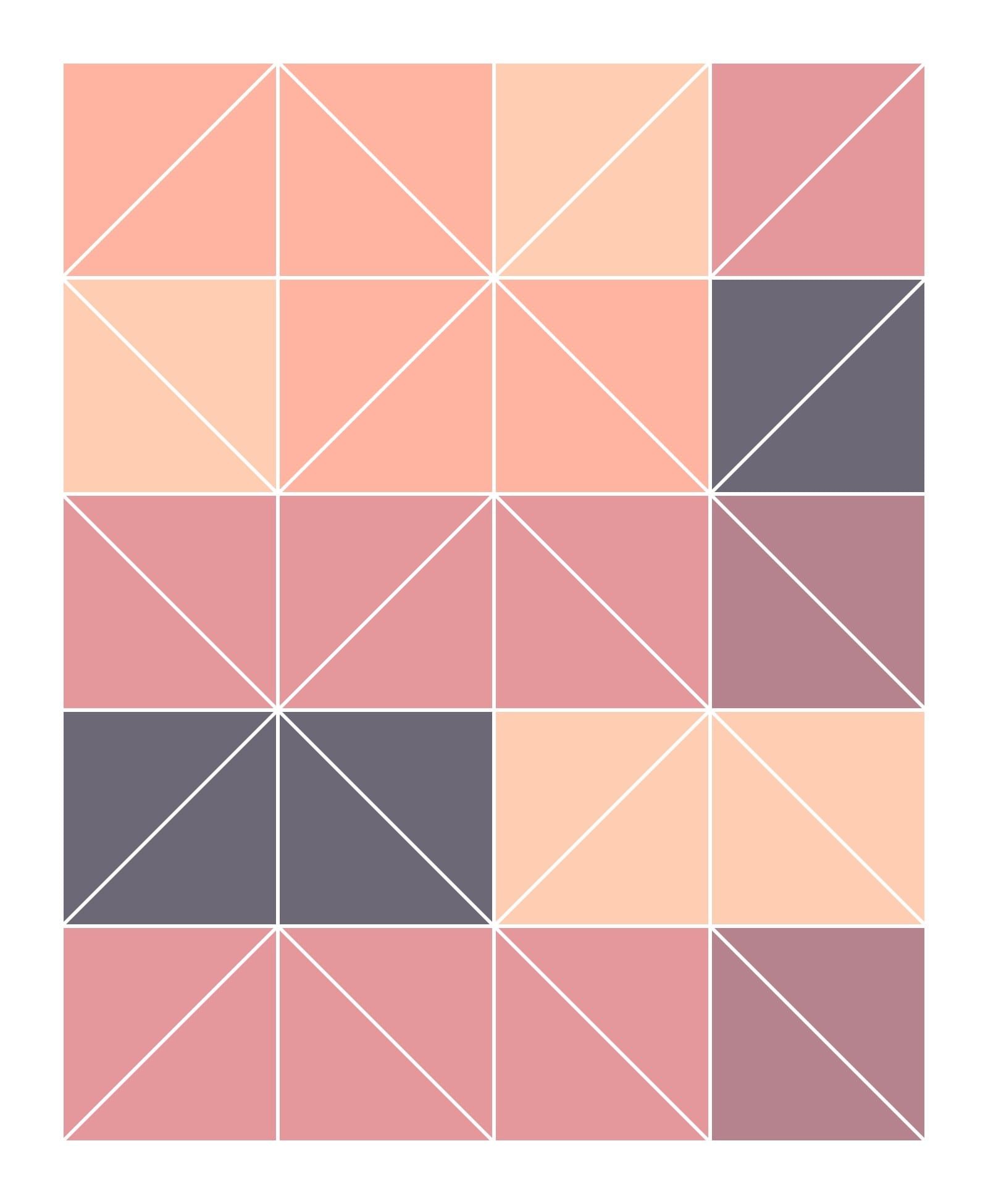
When diving into creative coding, one of the most powerful tools at your disposal is JavaScript classes. If you've been tinkering with code for a while, you've probably heard of object-oriented programming (OOP). Classes are at the heart of OOP, and they can help you organize and structure your code in a way that makes it more reusable, readable, and scalable—perfect for those generative art projects!
In this article, we'll break down what JavaScript classes are, why they’re useful for creative coding, and how you can start using them in your projects today. Whether you're a beginner or looking to refine your skills, understanding classes will elevate your coding game.
What Are JavaScript Classes?
At its core, a class is like a blueprint for creating objects. In JavaScript, objects are collections of related data and functionality. Think of a class as a template—you define the structure and behavior of the objects that can be created from it. This is especially useful in creative coding when you want to manage complex elements like shapes, animations, or interactive components in your generative art.
Here's a simple example of a JavaScript class:
class Circle {
constructor(x, y, radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
draw() {
ellipse(this.x, this.y, this.radius * 2);
}
move(dx, dy) {
this.x += dx;
this.y += dy;
}
}
In this Circle
class, we’ve defined a blueprint for creating circle objects. Each circle has properties like x
, y
, and radius
, and methods like draw
and move
. You can create multiple instances of Circle
, each with its own position and size, but all sharing the same behavior.
Why Use Classes in Creative Coding?
Using classes can streamline your creative coding process in several ways:
- Reusability: Once you've created a class, you can reuse it across different projects. For instance, if you've made a class for handling particles in a particle system, you can easily integrate it into other projects without rewriting the code.
- Organization: Classes help keep your code organized, especially in larger projects. You can bundle related functions and data together, making it easier to manage and modify your code.
- Scalability: As your projects grow in complexity, classes allow you to scale your codebase efficiently. By encapsulating functionality within classes, you can add new features or tweak existing ones without breaking your entire project.
How to Create and Use a JavaScript Class
Let's take a deeper dive into creating and using JavaScript classes in your creative coding projects.
Defining a Class
A class in JavaScript is defined using the class
keyword followed by the class name. Inside the class, you define a constructor method and any other methods that your objects will need.
class Particle {
constructor(x, y, speed, direction) {
this.x = x;
this.y = y;
this.speed = speed;
this.direction = direction;
}
update() {
this.x += cos(this.direction) * this.speed;
this.y += sin(this.direction) * this.speed;
}
display() {
ellipse(this.x, this.y, 5, 5);
}
}
Here, the Particle
class has a constructor that initializes the particle's position, speed, and direction. The update
method moves the particle, and the display
method draws it on the screen.
Creating Objects (Instances) from a Class
Once you've defined a class, you can create objects from it—these objects are called instances. Here’s how you would create a few particles:
let particles = [];
for (let i = 0; i < 100; i++) {
particles.push(new Particle(random(width), random(height), random(1, 3), random(TWO_PI)));
}
This code snippet creates an array of Particle
instances with random positions, speeds, and directions.
Using Methods
To make your particles come to life, you’ll need to update and display them in your draw
loop:
function draw() {
background(0);
for (let particle of particles) {
particle.update();
particle.display();
}
}
Each particle’s update
method is called to move it, and display
is called to draw it. Notice how clean and organized the code remains, even as the complexity increases.
Extending Classes
In creative coding, you might find that some shapes or behaviors are similar but not identical. JavaScript classes support inheritance, allowing you to create new classes based on existing ones.
For example, you might have a basic Shape
class and then extend it to create more specific shapes like Rectangle
and Triangle
:
class Shape {
constructor(x, y) {
this.x = x;
this.y = y;
}
move(dx, dy) {
this.x += dx;
this.y += dy;
}
}
class Rectangle extends Shape {
constructor(x, y, width, height) {
super(x, y);
this.width = width;
this.height = height;
}
draw() {
rect(this.x, this.y, this.width, this.height);
}
}
The Rectangle
class extends the Shape
class, inheriting its properties and methods, while also adding a draw
method specific to rectangles. This approach makes your code more modular and easier to manage.
Practical Applications in Creative Coding
Now that you understand the basics of classes, let’s look at how they can be applied in real-world creative coding projects.
1. Particle Systems
Classes are perfect for managing complex systems like particles. You can create a Particle
class and then use it to generate hundreds or thousands of particles, each behaving according to the rules you define in the class.
2. Interactive Art
If you're building interactive art pieces, classes can help you manage user interactions. For example, you could create a Button
class to handle different buttons on your canvas, each responding to clicks or other events in a consistent way.
3. Procedural Generation
For those into generative art, classes can help you create more sophisticated and reusable code. For instance, you might create a Tree
class to generate procedural trees, with each instance representing a unique tree.
Conclusion
Understanding and utilizing JavaScript classes can take your creative coding to the next level. By organizing your code into classes, you’ll make it more efficient, readable, and scalable, allowing you to focus on the creative aspects of your work.
Whether you're building particle systems, interactive art, or generative landscapes, JavaScript classes provide the structure and flexibility you need. So, dive in, experiment, and see how classes can transform your coding projects!
And remember, the more you practice, the more intuitive classes will become. Happy coding!
In this article, we've covered the fundamentals of JavaScript classes and their practical applications in creative coding. Be sure to explore other articles on our blog for more tips, tutorials, and insights into the world of generative art and creative coding with JavaScript.